What are Operators in C++? Types | Syntax | Implementation
Have you ever felt how it happens when you write a simple syntax of a = 5 + 4; on C or C++, you get the output 9? For this entire addition, subtraction, multiplication and division, you don’t need to worry about the internal working process.
Well, what if I told you that even for +, -, * and /, there is written a separate program and we call them Operator.
An Operator is defined as the set of basic functionalities like addition, multiplication, subtraction, division, etc., which help perform specific mathematical and logical computations on the Operands.
In other words, we can say that an operator operates the operands. Look into this picture, and you will get a clear understanding.
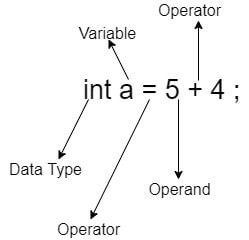
Operators are defined into 2 categories:
- Pre-defined Operators
- User-defined Operators
Pre-defined Operators are those which have been previously defined or written by the programmer and come with the installed compiler, whereas a User defines User-defined Operators and they are basically called Operator Overloading.
Types of Operators in C/C++
Operators are of 4 types:
Arithmetic Operator
Arithmetic operators in C++ include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). These operators are used to perform basic arithmetic operations on numerical values within C++ programs. Additionally, the increment (++) and decrement (–) operators are also available for use in C++ to conveniently modify numerical values.
Relational Operator
This operator, denoted by symbols such as ==, !=, <, >, <=, and >=, allows programmers to compare two values or variables and evaluate their relationship. By using these operators effectively, developers can make decisions within their code based on certain conditions.
The relational operator provides various outcomes based on the comparison made between two operands. For instance, the “==” operator checks if two values are equal; if they are indeed equal, it returns a true value; otherwise, it returns false. Conversely, the “!=” operator determines whether two values are not equal to each other. Similarly, the “<” and “>” operators check if one value is less than or greater than another respectively.
Logical Operator
The Logical Operators combine two or more Conditions or Constraints or complement the evaluation in the original condition. These are boolean type values and return either True or False to a given condition. For example:
&& is called as AND operator, and || is called as OR operator. These are similar to logic gates operation.
a && b returns true when both a and b are true, else false.
a || b returns true when either is true and false if both are false.
Bitwise Operator
Bitwise operators are used to perform bit-level operations on the operands. The operator first converts the operands into Bitwise level or binary representations of numbers and then performs the logical operations. & is called bitwise AND, and | is called bitwise OR. For example:
2 & 4, Here 2 is converted into a binary number as 0010 and 4 is converted into a binary number as 0100. So, 0010 & 0100 give 0
0010 | 0100 gives 0110 as the output and 6 as the number
Assignment Operator
The assignment operator is used to assign values to a variable. It is represented by ‘ = ’. For example, a = 5 + 5 ; assigns a 9 value to a variable.
‘ += ‘ or ‘-=’ are also called assignment operators. They first perform the operation and then assign the values. For example,
a += 5 is represented as “ a = a + 5”
a -= 5 is represented as “ a = a – 5”
Other Operators
There are some other operators which are also frequently used. These are basically,
Ternary Operator or Conditional Operator ( ? ): It is used to evaluate a condition like if else. For example, (conditional expression )? (option 1) : (option 2)
Option 1 is returned when a condition is true, else Option 2 is returned when the condition is false.
5 > 9 ? True : False
Answer is False
Sizeof (): Operator is used to give the operand’s size or length. It is a unary operand and takes an argument as an unsigned integral type, denoted by size_t.
For example, sizeof(8) integer gives 4 bytes. (Depends upon OS also)
Comma Operator (,): This operator, represented by a comma symbol (,), serves multiple purposes and can be used in a variety of ways to enhance code functionality. One common use of the comma operator is as a separator, allowing multiple expressions to be evaluated within a single statement. This can help streamline code and make it more concise.
Also Read: What are Loops in C and C++ Programming
Switch Statement in C and C++ ? Where to use Switch Case?