What are Loops in C and C++ Programming
Loops in C and C++ programming are constructs used to repeat a block of code multiple times. They provide a powerful mechanism to execute statements or perform certain operations repeatedly until a specific condition is met.
The Need for Loops
Have you ever thought that if you want to display 100 times message like “hello” on screen, or if you want a function/ part of the program to be executed several times, then without typing that many times you can go through Loop for the solution?
These are also referred to as repetitive tasks. As we know, when the process is repeated several times in a loop, the execution time will be reduced significantly. This kind of programming is required in various games, multimedia applications, etc. In all these applications, the loop is used to run the same program multiple times. Therefore, we can say that the loop is the best component for running a program multiple times.
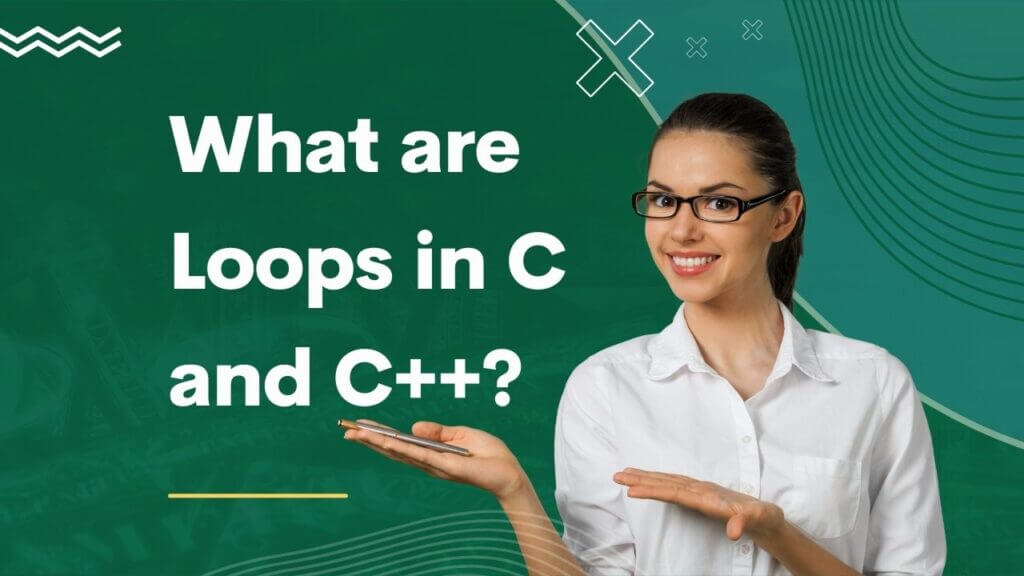
Also Read: What is an array in C/C++? – Basic Guide to C++
What are Operators in C++? Types | Syntax | Implementation
Loop in C/C++ is defined as a process of repeating the same task as per the requirement. A counter variable is always present there, and the program executes until the counter variable satisfies the condition. Loops are defined in two main types:
- Entry Controlled Loop
- Exit Controlled Loop
Entry-Controlled Loop: An entry-controlled loop is a type of loop construct where the loop condition is checked before executing the loop body. If the condition evaluates to true, the loop body is executed; otherwise, the loop is terminated and the program continues with the next statement after the loop.
For example,
Syntax in for loop
for ( initializer ; condition ; iteration){
}
//For loop
#include<iostrem>
using namespace std;
int main()
{
int i=0;
for( i=0 ; i<10 ; i++)
{
cout<<”Hello”<<endl;
}
return 0;
}
// while loop
Syntax :
initialization
while ( condition )
{
increment;
}
Example
int i=0;
while ( i < 10 )
{
cout<<”hello”<<endl;
i++;
}
Exit Controlled Loop: Here the condition is checked at the end of the loop.
For example, do – while () loop
Syntax
initialization
do {
iteration
}while (condition);
Even if the condition is false, the expression is executed at least once.
For example,
int i = 0;
do {
i++;
cout<<”Hello”<<endl;
} while ( i < 10 )
Infinite Loop
A loop which continues to run forever is known as infinite loop. For example,
for ( ; ; )
{
cout<<”Hello”<<endl;
}
Or
int i = 0;
while ( i ==0 )
{
cout<<”Hello”<<endl;
}
Conclusion
So we can create a simple loop for the given task and then exit the loop when the given task completes. Loops are used to execute a block of code at least once. So, loops are an essential concept in programming. C/C++ has the concept of while loops. A loop in C/C++ consists of a header block and a body block. The header block contains the condition or loop control statement. When the condition is true, the body block is executed. If the condition is false, the body block is not executed. Loops in C/C++ also come with different statements that are used to control the loop.